使用原生js写一个简单的注册登录页面
目录
1.首先是我们的注册页面
这是我们的html骨架
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>注册</title>
</head>
<link rel="stylesheet" href="./css/common.css">
<style>
body {
width: 100%;
height: 100%;
background-image: url(./img/RE53tTA.jfif);
background-repeat: no-repeat;
background-size: cover;
}
</style>
<body>
<div class="header">
<h2>注册</h2>
</span>
<label for="username"><span>用户名:</span><input type="text" id="use"></label>
<br>
<label for="password"><span>密码:</span><input type="password" id="pwd"></label>
<br>
<label for="password"><span>确认密码 </span><input type="password" id="pwd2"> </label>
<br>
<div>
<button onclick="login()">注册</button>
<button><a href="./登录.html">去登录</a></button>
</div>
</div>
</body>
</html>
这是css样式
* {
margin: 0;
padding: 0;
}
a {
text-decoration: none;
color: #fff;
font-size: 18px;
}
.header {
width: 400px;
height: 450px;
background: rgba(0, 0, 0, 0.2);
border-radius: 14px;
display: flex;
flex-direction: column;
margin: 100px 0 0 200px;
padding: 20px;
}
.header h2 {
font-size: 24px;
margin-top: 15px;
color: rgba(255, 255, 255, 0.7);
}
.header > label {
margin-top: 40px;
width: 350px;
display: flex;
align-items: center;
justify-content: space-between;
}
.header > label span {
font-size: 24px;
color: #fff;
}
.header > label input {
border-radius: 20px;
border: 1px solid #ccc;
padding: 0 20px;
background-color: rgba(255, 255, 255, 0.6);
box-sizing: border-box;
outline: none;
width: 240px;
height: 30px;
font-size: 18px;
}
.header > div {
margin-top: 30px;
display: flex;
justify-content: space-around;
width: 325px;
}
.header > div button {
width: 100px;
height: 30px;
background: rgba(0, 0, 0, 0.5);
border: none;
border-radius: 12px;
font-size: 18px;
color: #fff;
}
下面就是我们注册的js封装了
这里的函数调用直接写到了html里面的button事件上面了
<script>
//获取用户名;
let useA = localStorage.getItem('username');
console.log(useA);
//封装注册方法
function login() {
//获取事件的value
let username = document.querySelector('#use').value;
let password = document.querySelector('#pwd').value;
//这里调用存入本地的数据
Date(username, password);
}
//将数据存入本地
function Date(username, password) {
localStorage.setItem(username, JSON.stringify({
username,
password,
tag: false
}))
if (username === '' || password === '') {
alert('请先注册');
}
//判断两次密码是否一样
else if (pwd.value != pwd2.value) {
//不一样的话重新输入
alert('两次输入密码不一样 请重新输入');
} else {
//一样则提示注册成功
alert('注册成功 ! ! !')
}
}
</script>
这就是我的注册页面 ,当然各位要是觉得不好看也可以换成自己喜欢的图片;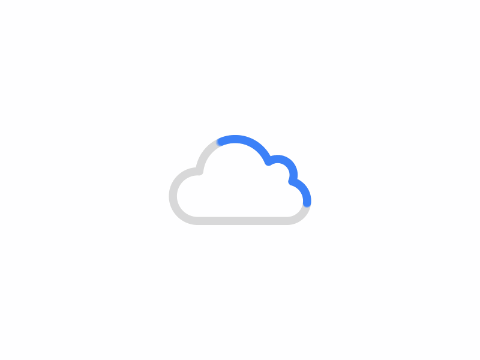
2.登陆页面
到这里当然要写我们的登录页面了,从注册页面获取数据 然后调用
html样式
<body>
<div class="header">
<h2>登录</h2>
<label for="username"><span>用户名:</span><input type="text" id="use"></label>
<br>
<label for="password"><span>密码:</span><input type="password" id="pwd"></label>
<br>
<div class="pwsd">
<input type="checkbox" id="cbx"><span>记住密码</span>
</div>
<div class="del">
<button onclick="login()">登录</button>
<button><a href="./注册.html">去注册</a></button>
</div>
</div>
</body>
css样式
<style>
a {
text-decoration: none;
color: #fff;
}
body {
width: 100%;
height: 100%;
background-image: url(./img/RE53r3l.jfif);
background-repeat: no-repeat;
background-size: cover;
}
.header {
width: 400px;
height: 450px;
background: rgba(0, 0, 0, .2);
border-radius: 14px;
display: flex;
flex-direction: column;
margin: 100px 0 0 200px;
padding: 20px;
}
h2 {
font-size: 24px;
color: #fff;
}
label {
margin-top: 40px;
width: 350px;
display: flex;
align-items: center;
justify-content: space-between;
}
label>span {
font-size: 24px;
color: #fff;
}
label>input {
border-radius: 20px;
border: 1px solid #ccc;
padding: 0 20px;
background-color: rgba(255, 255, 255, .6);
box-sizing: border-box;
outline: none;
width: 240px;
height: 30px;
font-size: 18px;
}
.del {
margin-top: 30px;
display: flex;
justify-content: space-around;
width: 325px;
}
.pwsd {
display: flex;
margin-top: 45px;
}
.pwsd>input {
width: 24px;
height: 24px;
}
.pwsd>span {
font-size: 18px;
color: #fff;
margin-left: 20px;
}
button {
width: 100px;
height: 40px;
background: rgba(0, 0, 0, .6);
border: none;
border-radius: 12px;
font-size: 18px;
color: #fff;
}
</style>
js样式
<script>
let use = document.getElementById('use');
let pwd = document.getElementById('pwd');
let cbx = document.getElementById('cbx');
function login() {
//登录
//取出本地的用户的值
let dateUse = localStorage.getItem(use.value);
console.log(dateUse)
let dateObj = JSON.parse(dateUse);//将取出的值转化为对象
console.log(dateObj);
if (use.value == dateObj.username && pwd.value == dateObj.password) {
alert('登陆成功');
dateObj.tag = cbx.checked;
localStorage.setItem(use.value, JSON.stringify(dateObj));//再将取出的值转化为字符串类型
location.href = 'https://www.baidu.com';//登录成功则跳转到百度页面
} else {
alert('用户名或者密码错误')
}
}
use.onblur = function () {//用户失去焦点事件s
//取出用户的值
let res = localStorage.getItem(use.value);
//将用户值转化为对象
res = JSON.parse(res);
if (res != null && res.tag) {//本地是否有该账号
cbx.checked = true;
pwd.value = res.password;
}
}
</script>
样品展示:
本图文内容来源于网友网络收集整理提供,作为学习参考使用,版权属于原作者。
THE END
二维码